Unfortunately TYPO3 doesn’t have a battle hardened ready to go REST API. Fortunately however this article will save you a load of time with a simple step by step guide to transform your TYPO3 application into an all singing and dancing REST API.
Why a REST API for TYPO3?
It’s worth considering why we would actually want to use a REST API for TYPO3. Let’s first look at some important concepts around REST API and how they can be advantageous for our web application.
Here are the key options for a REST API request:
- GET — The most common option, returns some data from the API based on the endpoint you visit and any parameters you provide
- POST — Creates a new record that gets appended to the database
- PUT — Looks for a record at the given URI you provide. If it exists, update the existing record. If not, create a new record
- DELETE — Deletes the record at the given URI
- PATCH — Update individual fields of a record
Let’s think of a REST API as a door into the database or in our case a door into our TYPO3 backend content. What will receive at that door is a Json response of data of whatever you have requested.
This will allow us to be free of the TYPO3 template system and we now build our websites with fast modern front end technologies such React or Vue.js, indeed we could even power a phone app from our TYPO3 backend.
Sounds kind of cool hah, so let’s going.
Install the Extbase Yaml Routes extension
There are quite a few extensions for creating a REST API in TYPO3. This one, Extbase Yaml Routes, gives a very simple solution which can easily be built on.
So go ahead and install and enable the Extbase Yaml Routes extension in your TYPO3 backend. You can do this with the following composer command.
composer req lms/routes
Install your site package extension
If your TYPO3 application hasn’t already have a site package extension go ahead and add one. This site package extension generator is recommended for a quick set up.
Create a test API Endpoint
Ok, time for the exciting part, let’s build our first API endpoint.
Configure the route
It’s TYPO3 of course so lets get configuring. In your site package create the following file.
my_package/Configuration/Routes.yml
Now in that the file lets configure a route.
api_test:
path: api/test
controller: MyPackage\Controller\TestApiController::test
methods: GET
format: json
defaults:
plugin: Testroute
We have named our route ‘api_test’. Let’s now go through the above settings.
Name the path of your API Endpoint
The path setting allow us to freely define the path of the API endpoint. We have named this one /api/test, we could if we wanted name it /myapi/v2/dogs/labradors
We will revisit this later but you can have a look here for best API REST route naming conventions.
Set up the controller that will return the response
The controller setting points to the method in the controller class that will return the json data for our endpoint. Let’s create that now.
Create the following TestApiController class that extends the Extbase ActionController
my_package/Classes/Controller/TestApiController.php
And inside that let’s write the following method.
public function testAction(): string
{
return json_encode(['labrador','pitbull','sheepdog']);
}
Obviously later we will be using the above methods for returning database queries and TYPO3 content etc.
Set the REST method
Here we can set the method. Remember we talked about these earlier. In our test we only want to get some data so we will use the GET method.
Create a plugin for the route
We need to create a TYPO3 plugin that will hook up the configured route and the controller. This is added in the following file.
my_package/ext_localconf.php
And add the following code, paying attention to names of your own classes and functions.
\TYPO3\CMS\Extbase\Utility\ExtensionUtility::configurePlugin(
'Vendor.my_package',
'Testplugin',
[
\MyPackage\Controller\TestApiController::class => 'test',
],
[]
);
That’s the very basics and now you have a callable API endpoint from your TYPO3 website. You can test the route directly in the browser or better still test it out in Postman.
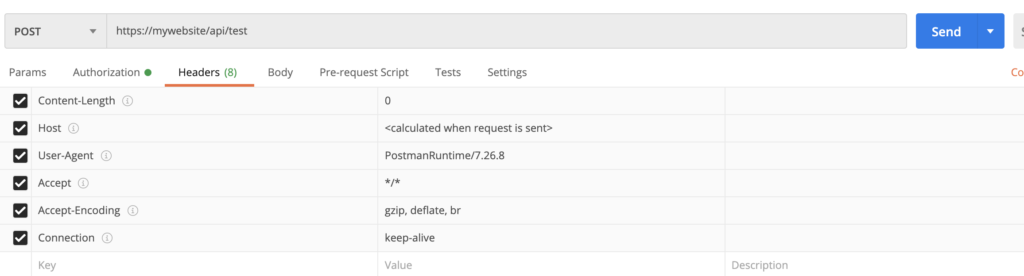
So the actual seemingly daunting process of turning a TYPO3 was done with just some simple steps and the excellent work from the YAML routes extension which takes advantage of the middleware layer introduced in TYPO3 version 10.
Leave a Reply